EMAIL SUPPORT
dclessons@dclessons.comLOCATION
USPython Integers basics
Below are some base point used in Python for integers
Integers are created in python using following rules
- Python is a case sensitive language
- Integer’s starts with alphabet or an underscore, followed by 0 or more alphabets letters, including _, digits.
- Keywords are not used as integer in Python.
Keywords
- All keywords used in Python are case sensitive
- Python uses 33 keywords, some of them are given below
- False,del,for,in,or,while,break,elif,from,is,pass,with, true,else,class,raise,and,continue,except,if,returm,as.
Some of the keywords can also be obtained by below statement
- Import < “keyword”>
- Print(keyword.kwname)
Python Types
Python has following built-in types, mentioned below. We will see some of them by some example
Basic Types: Int, float, complex, bool, string, bytes
Let’s understand some of them with some example.
Int:
These can be expressed in binary, decimal, octal, and hexadecimal
- Binary: 0 or 1
- Decimal: 12.34, -23.45, 31.4e2
- Complex : 3+2j, 1+4j
- Bool: True or False
- String: “dclessons”
Container Type:
it can be defined as list, tuple, set, dict
- List: it contains list of similar or dissimilar items. [1,2,3,4,5,6,7,8,] , [‘alo’,’bhi’,12,45,]
- Tuple: it contains collection of static items. (‘dclessons’, 45, ‘let learns python’)
- Set: It is collection of unique values. { 10,20,30,40} , {‘dclessons’, 23,45}
Variables:
In python, we don’t need to define the type of variable. As soon as we assign the value to variable, it inherits its type based on type of values.
Example:
- A=10: a inherits the int type
- A= ‘dc’: A inherits its type as string
- A= 10.34: A inherits its type as float
Multiple variable can also be assigned in Python
Example:
- A = 11; dc=23.2; add=’NZ’
- A,dc, add= 11,23,2,’NZ’
- A = x = y = z = 10
We can also check the type of variable by using built-in function type ()
A = 10.23
Print (type (A))
This command will return the value float. : <class 'float'>
Arithmetic Operators
Below are list of Arithmetic operator used in python
+ , - , * , / , % , // , **
Let’s see what the function of some of them:
%: always gives the remainder 1.
5%2 = 1
Let’s see another feature of %, Operation of X%Y can be seen as x-(y*(x//y))
Print (10%3) = 10-(3*(10//3)) = 1
Like wise
- Print (-10%3) = 2
- Print(3%10)= 3
- Print(-3%10) = 7
**: Yields for exponential values
2**3 = 2 raised to power 3 = 8
//: It returns quotient 1 after discarding fraction part.
- 5//2 = 1
- Print (10//3) = 3
- Print 10//-3) = 3
- Print (3//10) = 0
- Print (-3/10) = -1
Example 1: Lets learn some exercise for operator
print(5/6) print(4%5) print(5//6) print(5**4) ========================= Result 0.833 4 0 625
Example 2:
a = 20; b = 25 ; c = 10; d= 20; e=5; f=4; g=10 x=a+b-c y=d**e z=f%g print(x,y,z) =========================== Result 35 3200000 4
In-Place Operators:
In-Place operator are those operator which are used mostly for shortcuts. These include +=, *=, %=, //=, **=.
Let’s see by example:
- A** = 3 is same as A= A**3
- B%=5 is same as B= B%5
Operator Precedence
Operator Precedence As we know that there are math’s operator, and they follow some precedence, so let’s see how they take precedence n python
When multiple operator are used in any arithmetic, it is used on the basis of priority based on rule said as (PEMDAS).
- (): Parentheses
- **: Exponentiation
- *, /, //, %: multiplication, division
- +, - : Addition, Subtraction
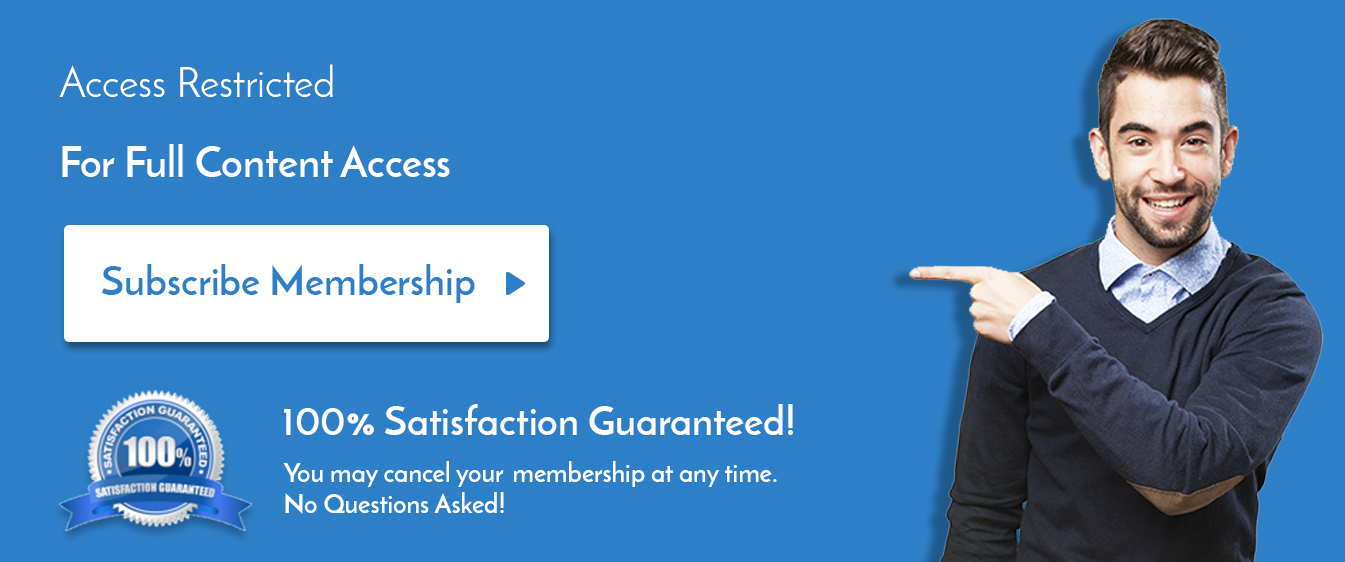
LEAVE A COMMENT
Please login here to comment.